Read Array of Dictionaries From Txt Python
Overview
When y'all're working with Python, y'all don't need to import a library in order to read and write to a file. It'due south handled natively in the language, albeit in a unique mode. Below, we outline the simple steps to read and write to a file in Python.
The commencement thing yous'll need to do is use the built-in python open file part to get a file object .
The open function opens a file. It'due south uncomplicated. This is the outset step in reading and writing files in python.
When you use the open up function, it returns something chosen a file object . File objects contain methods and attributes that can be used to collect information near the file yous opened. They tin can also be used to manipulate said file.
For example, the mode aspect of a file object tells you which mode a file was opened in. And the name attribute tells y'all the name of the file.
You must empathize that a file and file object are ii wholly separate – even so related – things.
File Types
What you lot may know as a file is slightly different in Python.
In Windows, for example, a file can be any item manipulated, edited or created by the user/Os. That means files can be images, text documents, executables, and excel file and much more. Most files are organized by keeping them in private folders.
A file In Python is categorized every bit either text or binary, and the difference between the two file types is important.
Text files are structured equally a sequence of lines, where each line includes a sequence of characters. This is what y'all know as code or syntax.
Each line is terminated with a special character, chosen the EOL or End of Line grapheme. There are several types, only the about common is the comma {,} or newline graphic symbol. Information technology ends the electric current line and tells the interpreter a new one has begun.
A backslash character tin can too be used, and information technology tells the interpreter that the adjacent character – post-obit the slash – should be treated as a new line. This graphic symbol is useful when you lot don't want to start a new line in the text itself simply in the code.
A binary file is whatever type of file that is not a text file. Considering of their nature, binary files can only exist processed by an application that know or empathise the file'south structure. In other words, they must be applications that can read and translate binary.
Reading Files in Python
In Python, files are read using the open() method. This is one of Python'south built-in methods, made for opening files.
The open() role takes two arguments: a filename and a file opening style. The filename points to the path of the file on your estimator, while the file opening mode is used to tell the open() function how we plan to collaborate with the file.
By default, the file opening manner is set to read-only, pregnant nosotros'll only have permission to open up and examine the contents of the file.
On my computer is a folder called PythonForBeginners. In that binder are three files. I is a text file named emily_dickinson.txt, and the other 2 are python files: read.py and write.py.
The text file contains the following poem, written by poet Emily Dickinson. Maybe we are working on a poetry program and take our poems stored every bit files on the computer.
Success is counted sweetest
By those who ne'er succeed.
To comprehend a nectar
Requires sorest need.
Not one of all the Purple Host
Who took the Flag today
Can tell the definition
So clear of Victory
As he defeated, dying
On whose forbidden ear
The distant strains of triumph
Burst aching and clear.
Before nosotros can practise anything with the contents of the poem file, we'll need to tell Python to open up it. The file read.py, contains all the python code necessary to read the poem.
Any text editor can be used to write the lawmaking. I'm using the Atom lawmaking editor, which is my editor of option for working in python.
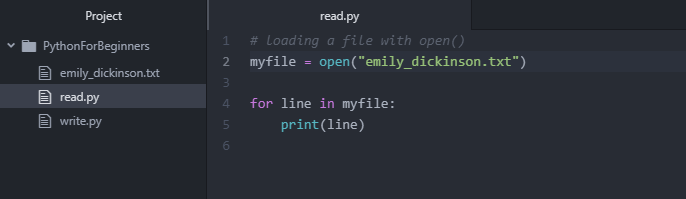
# read.py # loading a file with open() myfile = open("emily_dickinson.txt") # reading each line of the file and press to the console for line in myfile: print(line)
I've used Python comments to explain each step in the code. Follow this link to learn more well-nigh what a Python comment is.
The example to a higher place illustrates how using a simple loop in Python tin read the contents of a file.
When it comes to reading files, Python takes care of the heaving lifting behind the scenes. Run the script by navigating to the file using the Control Prompt — or Terminal — and typing 'python' followed by the name of the file.
Windows Users: Earlier you tin apply the python keyword in your Command Prompt, y'all'll demand to gear up upwards the environment variables. This should have happened automatically when yous installed Python, but in case it didn't, you lot may need to practice it manually.
>python read.py
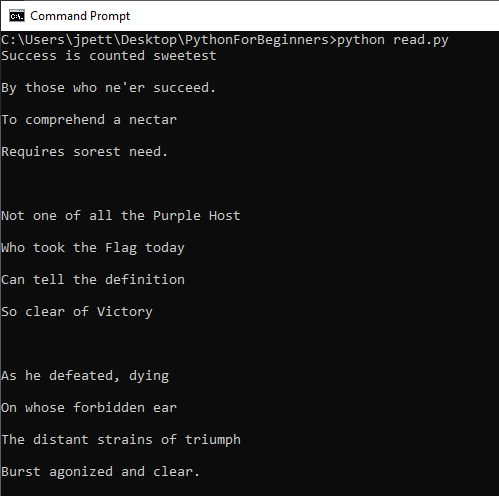
Data provided by the open up() method is commonly stored in a new variable. In this example, the contents of the poem are stored in the variable "myfile."
One time the file is created, we tin can use a for loop to read every line in the file and print its contents to the control line.
This is a very simple example of how to open a file in Python, but student'due south should be aware that the open() method is quite powerful. For some projects it will be the just matter needed to read and write files with Python.
Writing Files in Python
Earlier we can write to a file in Python, it must outset exist opened in a different file opening mode. Nosotros can do this by supplying the open up() method with a special argument.
In Python, write to file using the open() method. You'll need to pass both a filename and a special character that tells Python we intend to write to the file.
Add the following code to write.py. Nosotros'll tell Python to look for a file named "sample.txt" and overwrite its contents with a new message.
# open the file in write manner myfile = open up("sample.txt",'west') myfile.write("Hello from Python!")
Passing 'westward' to the open() method tells Python to open the file in write mode. In this mode, whatever data already in the file is lost when the new data is written.
If the file doesn't exist, Python will create a new file. In this instance, a new file named "sample.txt" volition exist created when the program runs.
Run the program using the Command Prompt:
>python write.py
Python can as well write multiple lines to a file. The easiest way to do this is with the writelines() method.
# open the file in write mode myfile = open up("sample.txt",'w') myfile.writelines("Hello Globe!","We're learning Python!") # shut the file myfile.close()
We can besides write multiple lines to a file using special characters:
# open the file in write mode myfile = open("poem.txt", 'west') line1 = "Roses are red.\n" line2 = "Violets are bluish.\n" line3 = "Python is great.\n" line4 = "And so are you.\northward" myfile.write(line1 + line2 + line3 + line4)
Using string chain makes information technology possible for Python to salvage text information in a variety of ways.
However, if we wanted to avert overwriting the data in a file, and instead suspend it or change it, we'd have to open the file using another file opening mode.
File Opening Modes
By default, Python will open up the file in read-only style. If we want to do anything other than simply read a file, we'll demand to manually tell Python what we intend to exercise with it.
- 'r' – Read Manner: This is the default mode for open() . The file is opened and a pointer is positioned at the beginning of the file's content.
- 'w' – Write Mode: Using this mode will overwrite any existing content in a file. If the given file does not exist, a new i volition be created.
- 'r+' – Read/Write Mode: Use this manner if you demand to simultaneously read and write to a file.
- 'a' – Suspend Mode: With this fashion the user tin append the data without overwriting whatsoever already existing data in the file.
- 'a+' – Append and Read Mode: In this mode you can read and append the information without overwriting the original file.
- 'x' – Exclusive Creating Mode: This mode is for the sole purpose of creating new files. Use this style if yous know the file to be written doesn't exist beforehand.
Note: These examples assume the user is working with text file types. If the intention is to read or write to a binary file type, an additional argument must be passed to the open() method: the 'b' graphic symbol.
# binary files need a special statement: 'b' binary_file = open("song_data.mp3",'rb') song_data = binary_file.read() # close the file binary_file.close()
Closing Files with Python
After opening a file in Python, information technology's of import to close it after you lot're done with it. Closing a file ensures that the programme can no longer admission its contents.
Close a file with the close() method.
# open a file myfile = open("poem.txt") # an assortment to store the contents of the file lines = [] For line in myfile: lines.suspend(line) # close the file myfile.close() For line in liens: impress(line)
Opening Other File Types
The open() method can read and write many dissimilar file types. We've seen how to open up binary files and text files. Python can also open images, allowing yous to view and edit their pixel data.
Before Python tin open up an image file, the Pillow library (Python Imaging Library) must be installed. It's easiest to install this module using pip.
pip install Pillow
With Pillow installed, Python tin open image files and read their contents.
From PIL import Image # tell Pillow to open the image file img = Image.open("your_image_file.jpg") img.evidence() img.shut()
The Pillow library includes powerful tools for editing images. This has fabricated information technology ane of the most popular Python libraries.
With Argument
You can likewise work with file objects using the with argument. Information technology is designed to provide much cleaner syntax and exceptions handling when you are working with code. That explains why it's practiced practice to use the with statement where applicative.
1 bonus of using this method is that any files opened will be closed automatically afterwards you are done. This leaves less to worry about during cleanup.
To use the with statement to open a file:
with open("filename") as file:
Now that you understand how to call this argument, allow's take a expect at a few examples.
with open up("poem.txt") as file: data = file.read() do something with data
You can also telephone call upon other methods while using this statement. For case, you can do something like loop over a file object:
due westith open up("poem.txt") every bit f: for line in f: print line,
You'll also notice that in the above case we didn't use the " file.close() " method because the with statement volition automatically call that for usa upon execution. It really makes things a lot easier, doesn't it?
Splitting Lines in a Text File
As a final example, let'southward explore a unique office that allows y'all to split the lines taken from a text file. What this is designed to do, is split the cord independent in variable data whenever the interpreter encounters a space character.
But just because we are going to use information technology to dissever lines later a space character, doesn't hateful that's the only mode. You can actually split up your text using any character you wish – such as a colon, for instance.
The code to exercise this (also using a with statement) is:
with open up( " howdy.text ", "r") as f: information = f.readlines () for line in information: words = line.divide () impress words
If y'all wanted to use a colon instead of a infinite to split your text, you would simply change line.split() to line.separate(":").
The output for this will be:
["hello", "globe", "how", "are", "you", "today?"] ["today", "is", "Saturday"]
The reason the words are presented in this fashion is because they are stored – and returned – as an array. Be sure to call back this when working with the split office.
Conclusion
Reading and writing files in Python involves an understanding of the open() method. Past taking advantage of this method's versatility, it'southward possible to read, write, and create files in Python.
Files Python can either be text files or binary files. It's also possible to open and edit paradigm data using the Pillow module.
One time a file's data is loaded in Python, in that location'south about no end to what can be done with it. Programmers ofttimes piece of work with a big number of files, using programs to generate them automatically.
Every bit with any lesson, in that location is only and then much that can exist covered in the space provided. Hopefully you've learned plenty to go started reading and writing files in Python.
More Reading
Official Python Documentation – Reading and Writing Files
Python File Treatment Crook Sheet
Recommended Python Training
Course: Python three For Beginners
Over 15 hours of video content with guided instruction for beginners. Learn how to create real world applications and chief the basics.
Source: https://www.pythonforbeginners.com/files/reading-and-writing-files-in-python
0 Response to "Read Array of Dictionaries From Txt Python"
Post a Comment